/* The following C++ Program Code will be used to implement the circular linked list. The user is asked first to create the circular linked list, & when he is done entering the element in the list , he will enter 0. After creation to the initial circular list, he is given the option of insertion, deletion & display of the circular linked list thus created. Also provided with the program exit option. */
#include<iostream>
#include<stdlib.h>
using namespace std;
template<class T>
class node
{
public:
T data;
node<T> *next;
};
template<class T>
class list
{
private:
node<T> *first;
public:
list();
~list();
void create();
void del();
void ins();
void disp();
};
template<class T>
list<T>::list()
{
first=NULL;
}
template<class T>
list<T>::~list()
{
node<T> *next;
while(first)
{
next=first->next;
delete first;
first=next;
}
}
template<class T>
void list<T>::create()
{
T a;
node<T> *cur,*ptr;
first=NULL;
cout<<"\nEnter data : ";
cin>>a;
while(a)
{
cur=new node<T>;
cur->data=a;
cur->next=NULL;
if(first==NULL)
first=cur;
else
ptr->next=cur;
ptr=cur;
cout<<"\nEnter data : ";
cin>>a;
}
ptr->next=first;
}
template<class T>
void list<T>::ins()
{
node<T> *cur,*ptr,*exmp;
T ele;
char ch;
ptr=exmp=first;
cur=new node<T>;
cout<<"\nEnter data : ";
cin>>cur->data;
cur->next=NULL;
cout<<"\nDo you want to insert at first [y/n] ? : ";
cin>>ch;
if(ch=='Y'||ch=='y')
{
while(ptr->next!=first)
{
ptr=ptr->next;
}
cur->next=first;
first=cur;
ptr->next=first;
}
else
{
cout<<"\nAfter which element you want to insert ? : ";
cin>>ele;
do
{
if(exmp->data==ele)
{
cur->next=exmp->next;
exmp->next=cur;
}
exmp=exmp->next;
}while(exmp!=first);
}
}
template<class T>
void list<T>::del()
{
T ele;
char ch;
node<T> *ptr,*ptr1;
ptr=ptr1=first;
cout<<"\nDo you want to delete the first element [y/n] ? : ";
cin>>ch;
if(ch=='y'||ch=='Y')
{
while(ptr->next!=first)
{
ptr=ptr->next;
}
first=ptr1->next;
ptr->next=first;
}
else
{
cout<<"\nWhich element to delete? : ";
cin>>ele;
do
{
if(ptr1->next->data==ele)
{
ptr1->next=ptr1->next->next;
break;
}
ptr1=ptr1->next;
}while(ptr1!=first);
}
}
template<class T>
void list<T>::disp()
{
node<T> *ptr;
ptr=first;
do
{
cout<<ptr->data<<"---->";
ptr=ptr->next;
}while(ptr!=first);
}
int main()
{
int n;
list <int> x;
cout<<"\nEnter the elements to create initial list (Enter 0 when done)\n";
x.create();
do
{
cout<<"\n 1.Insert Element \n2.Delete Element \n3.Display Element \n4.Exit \n";
cout<<"\nEnter option : ";
cin>>n;
switch(n)
{
case 1: x.ins();
break;
case 2: x.del();
break;
case 3: x.disp();
break;
case 4: exit(0);
break;
default: cout<<"\nWrong Choice Entered!";
}
}while(n<=4);
system("pause");
return 0;
}
Screenshot:
#include<iostream>
#include<stdlib.h>
using namespace std;
template<class T>
class node
{
public:
T data;
node<T> *next;
};
template<class T>
class list
{
private:
node<T> *first;
public:
list();
~list();
void create();
void del();
void ins();
void disp();
};
template<class T>
list<T>::list()
{
first=NULL;
}
template<class T>
list<T>::~list()
{
node<T> *next;
while(first)
{
next=first->next;
delete first;
first=next;
}
}
template<class T>
void list<T>::create()
{
T a;
node<T> *cur,*ptr;
first=NULL;
cout<<"\nEnter data : ";
cin>>a;
while(a)
{
cur=new node<T>;
cur->data=a;
cur->next=NULL;
if(first==NULL)
first=cur;
else
ptr->next=cur;
ptr=cur;
cout<<"\nEnter data : ";
cin>>a;
}
ptr->next=first;
}
template<class T>
void list<T>::ins()
{
node<T> *cur,*ptr,*exmp;
T ele;
char ch;
ptr=exmp=first;
cur=new node<T>;
cout<<"\nEnter data : ";
cin>>cur->data;
cur->next=NULL;
cout<<"\nDo you want to insert at first [y/n] ? : ";
cin>>ch;
if(ch=='Y'||ch=='y')
{
while(ptr->next!=first)
{
ptr=ptr->next;
}
cur->next=first;
first=cur;
ptr->next=first;
}
else
{
cout<<"\nAfter which element you want to insert ? : ";
cin>>ele;
do
{
if(exmp->data==ele)
{
cur->next=exmp->next;
exmp->next=cur;
}
exmp=exmp->next;
}while(exmp!=first);
}
}
template<class T>
void list<T>::del()
{
T ele;
char ch;
node<T> *ptr,*ptr1;
ptr=ptr1=first;
cout<<"\nDo you want to delete the first element [y/n] ? : ";
cin>>ch;
if(ch=='y'||ch=='Y')
{
while(ptr->next!=first)
{
ptr=ptr->next;
}
first=ptr1->next;
ptr->next=first;
}
else
{
cout<<"\nWhich element to delete? : ";
cin>>ele;
do
{
if(ptr1->next->data==ele)
{
ptr1->next=ptr1->next->next;
break;
}
ptr1=ptr1->next;
}while(ptr1!=first);
}
}
template<class T>
void list<T>::disp()
{
node<T> *ptr;
ptr=first;
do
{
cout<<ptr->data<<"---->";
ptr=ptr->next;
}while(ptr!=first);
}
int main()
{
int n;
list <int> x;
cout<<"\nEnter the elements to create initial list (Enter 0 when done)\n";
x.create();
do
{
cout<<"\n 1.Insert Element \n2.Delete Element \n3.Display Element \n4.Exit \n";
cout<<"\nEnter option : ";
cin>>n;
switch(n)
{
case 1: x.ins();
break;
case 2: x.del();
break;
case 3: x.disp();
break;
case 4: exit(0);
break;
default: cout<<"\nWrong Choice Entered!";
}
}while(n<=4);
system("pause");
return 0;
}
Screenshot:
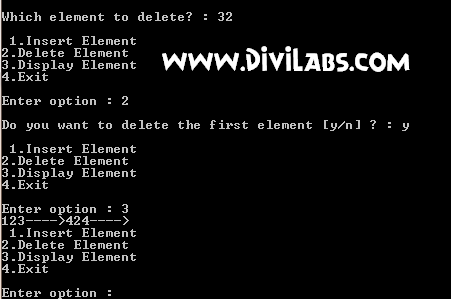
0 comments:
Post a Comment